The If/Else and the Switch(/Case) statements are pretty similar, but what are the differences? Which one should you use and when? This post will answer those curious questions for you. Before we begin, I suggest that you read this wiki page in case if you don't know about the Switch statement.
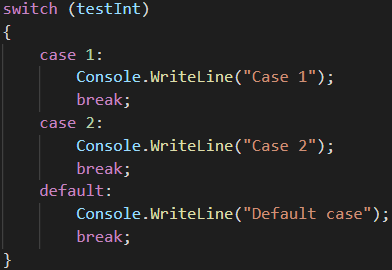
First off, I would like to start with the main difference. Unlike If/Else that can work with multiple variables and conditions in one statement, a Switch statement can only work with one variable and one condition, E.G. if a number is 3. However, this doesn't mean that you should prefer If/Else over switches every time.
I will explain this with the second difference, which is how If/Else and Switch works with their statements and "conditions".
With If/Else, each statement are evaluated sequentially. I will use this piece of code as an example.
int myInt = 1;
if (myInt == 1)
{
// Do something if myInt is 1
}
else if (myInt == 2)
{
// Do something if myInt is 2
}
else
{
// Do something if neither of the two conditions above are true
}
When you run this code, the (myInt == 1) condition is evaluated first, then the second one, and so forth.
Now here's a switch case version of the code above.
switch (myint)
{
case 1:
// Do something if myInt is 1
break;
case 2:
// Do something if myInt is 2
break;
default:
// Do something if neither of the two conditions above are true
break;
}
While very similar, switch case uses a "jump table" that it will use for selecting the path of execution depending on the value of the expression instead of evaluating one condition at a time. This means that if you have a lot of statements that are just "if this variable equals this then do that", you might want to use switch case as a faster alternative.
While switch case is a good choice for some uses, there are areas where If/Else is a better choice. The obvious one is when you have multiple conditions in one state meant, for example:
if (myInt > 0 && myInt < 10) //if myInt is more than 0 and less than 10
This is a condition that you cannot do using switch case(There are some hacky workarounds on StackOverflow but I won't touch on that topic) and have do be done using Ifs.
To conclude this post, the answer to the question in the title is.... it depends. If you only have a few statements and/or have to use multiple conditions or variables in one statement, you would want to use If/Else. If you have a lot of statements and only have to deal with one variable, switch case if for you.
And that's it for this post! Thanks to Microsoft's C# Doc for providing a few sample codes and GeeksforGeeks for the comparison between If/Else and switch case 👍
Comments